Table of Contents
Spring Boot is a widely used framework for developing enterprise web applications. By default, Spring Boot applications run on a specific port, usually 8080. However, there are some scenarios where we need to change the default port number, to avoid conflicts with other services and applications.
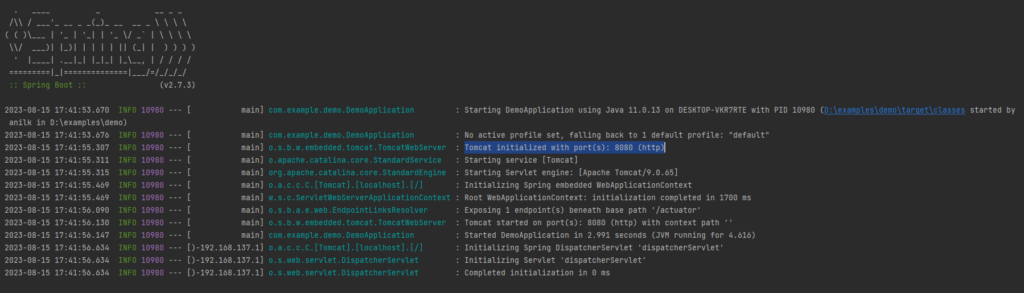
The default port (8080) is convenient for development purposes, but it might not be suitable for production environments or when dealing with multiple applications on the same server.
In this article, We will learn various ways to change the default port number in Spring Boot.
Change Spring Boot Default Port Number
1. Application Properties
One of the most straightforward methods to change the spring boot default port number is by modifying the application.properties
or application.yml file. This file is typically located in the src/main/resources
directory of your Spring Boot project. You can add or modify the following line to set a new port.
server.port=8081
server:
port : 8081
In this example, the application will now run on port 8081. Remember to restart your application for the changes to take effect.
2. Command Line Arguments
Another way to change the spring boot default port number is by passing it as a command-line argument when starting the Spring Boot application. Use the --server.port
option followed by the desired port number.
java -jar myapp.jar --server.port=9090
java -jar -Dserver.port=9090 myapp.jar
This approach allows you to easily switch port numbers without modifying the application’s configuration files.
3. Programmatically in Java Code
If you need more dynamic control over the port, you can configure it programmatically in your Java code. Create a configuration class that implements the WebServerFactoryCustomizer
interface
import org.springframework.boot.web.server.WebServerFactoryCustomizer;
import org.springframework.boot.web.server.ConfigurableWebServerFactory;
import org.springframework.stereotype.Component;
@Component
public class ServerPortCustomizer implements WebServerFactoryCustomizer<ConfigurableWebServerFactory> {
@Override
public void customize(ConfigurableWebServerFactory factory) {
factory.setPort(6060);
}
}
In this example, the application will start on port 6060. This method is particularly useful when you need more advanced logic to determine the port dynamically.
Order of Evaluation
- embedded server configuration
- command-line arguments
- property files
- Spring Boot Application configuration
Conclusion
In this article, we saw how to change the spring boot default port number. Changing the default port number in Spring Boot is essential when dealing with various deployment scenarios or port conflicts. Whether it’s through the application.properties file, command-line arguments, or Java code, Spring Boot provides several flexible methods to configure the port to suit your needs.
To access more articles on Spring Boot, we welcome you to explore our easy2excel.in website.